반응형
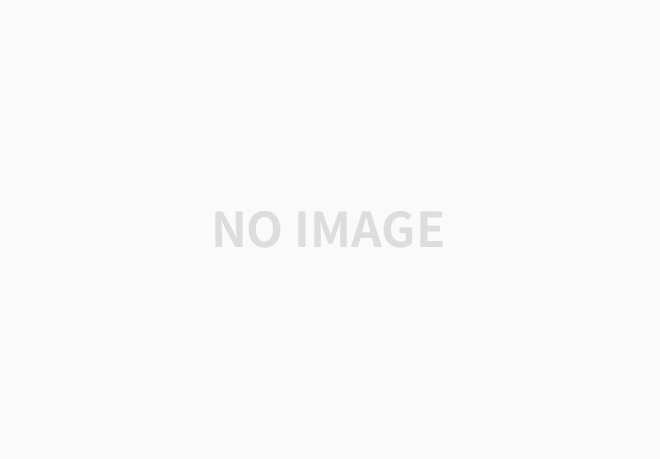
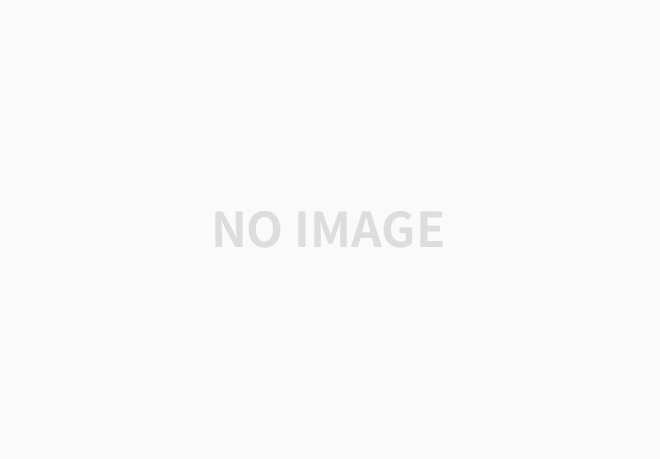
앱 내의 평점 유도 팝업
게임 평점 팝업을 띄우고 싶은 경우가 생깁니다. 이 점수는 실제로 앱 평가에도 많은 영향을 주고 참여도 및 점수가 높으면 앱이 조금이라도 더 노출될 확률이 생깁니다. 게임에 자연스럽게 오버레이가 되어 리뷰 요청을 하도록 기능을 넣을 수 있습니다.
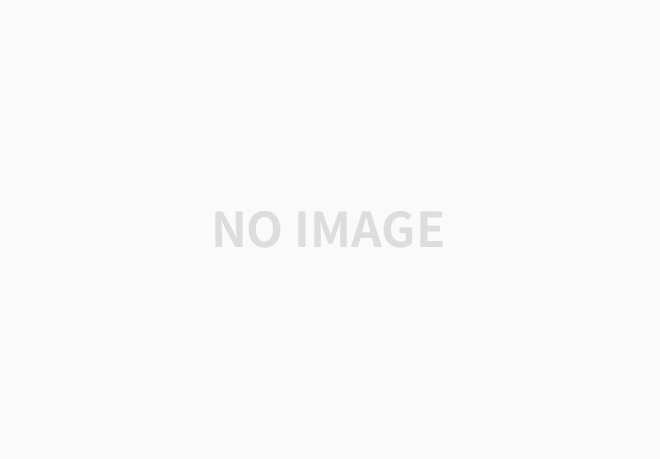
앱스토어 리뷰 평가의 경우 유니티에서 내장 함수가 있으며, 구글 플레이 스토어 리뷰 평가는 따로 API를 사용해야합니다. 아래의 기능을 사용하기 전에 Play Core 라이브러리를 요구하기에 설치해주셔야 합니다.
스크립트
using System;
using System.Collections;
using UnityEngine;
#if UNITY_ANDROID
using Google.Play.Review;
#endif
public class StoreReview : MonoBehaviour
{
#region ## Instance ##
/// <summary>
/// 코루틴 사용을 위한 인스턴스
/// </summary>
private static StoreReview _instance;
private static StoreReview Instance
{
get
{
if (_instance == null)
{
var go = new GameObject("Store Review");
_instance = go.AddComponent<StoreReview>();
}
return _instance;
}
}
#endregion
#region ## File I/O ##
private static bool? _isAlreadyReview = null;
/// <summary>
/// 리뷰 요청한 적이 있는지 체크
/// </summary>
private static bool IsAlreadyReview
{
get
{
if (_isAlreadyReview == null)
{
_isAlreadyReview = PlayerPrefs.GetInt("StoreReview", 0) == 1;
}
return _isAlreadyReview.Value;
}
set
{
if (_isAlreadyReview != value)
{
_isAlreadyReview = value;
PlayerPrefs.SetInt("StoreReview", value ? 1 : 0);
}
}
}
#endregion
/// <summary>
/// 리뷰 팝업 요청하기
/// </summary>
public static void Open()
{
if (!IsAlreadyReview)
{
// 파일 저장 하기
IsAlreadyReview = true;
#if UNITY_ANDROID
RequestGooglePlayStoreReview();
#elif UNITY_IOS
RequestAppStoreReview();
#endif
}
else
{
// 잦은 노출은 정책 위반 또는 리뷰 팝업이 등장하지 않기 때문에 1회 요청으로 한정
Debug.Log("이미 리뷰 평점 요청을 한 적이 있습니다.");
}
}
/// <summary>
/// 구글 플레이 스토어 리뷰 평가 요청
/// </summary>
private static void RequestGooglePlayStoreReview()
{
#if UNITY_ANDROID
Instance.StartCoroutine(RequestGooglePlayStoreReviewCoroutine(() =>
{
if (Instance != null)
{
Destroy(Instance.gameObject);
}
}));
#endif
}
/// <summary>
/// 구글 플레이 스토어 리뷰 평가 요청 코루틴
/// https://developer.android.com/guide/playcore/in-app-review/unity?hl=ko
/// </summary>
/// <returns></returns>
private static IEnumerator RequestGooglePlayStoreReviewCoroutine(Action onFinished)
{
// 구글 리뷰 가이드
// '할당량'
// - 우수한 사용자 환경을 제공하기 위해 Google Play는 사용자에게 리뷰 대화상자를 표시할 수 있는 빈도에 관한 시간제한 할당량을 적용합니다.
// - 이 할당량으로 인해 짧은 기간(예: 1개월 미만) launchReviewFlow 메서드를 두 번 이상 호출할 경우 대화상자가 표시되지 않을 수도 있습니다.
#if UNITY_ANDROID
ReviewManager reviewManager = new ReviewManager();
var requestFlowOperation = reviewManager.RequestReviewFlow();
yield return requestFlowOperation;
if (requestFlowOperation.Error != ReviewErrorCode.NoError)
{
Debug.LogError("requestFlowOperation" + requestFlowOperation.Error);
//OpenGooglePlayStorePage();
onFinished?.Invoke();
onFinished = null;
yield break;
}
PlayReviewInfo playReviewInfo = requestFlowOperation.GetResult();
var launchFlowOperation = reviewManager.LaunchReviewFlow(playReviewInfo);
yield return launchFlowOperation;
playReviewInfo = null; // Reset the object
if (launchFlowOperation.Error != ReviewErrorCode.NoError)
{
Debug.LogError("launchFlowOperation: " + launchFlowOperation.Error);
//OpenGooglePlayStorePage();
onFinished?.Invoke();
onFinished = null;
yield break;
}
Debug.Log("Open Review Popup");
#endif
onFinished?.Invoke();
onFinished = null;
yield break;
}
/// <summary>
/// 구글 플레이 스토어 페이지 열기
/// </summary>
private static void OpenGooglePlayStorePage()
{
#if UNITY_ANDROID
Application.OpenURL($"market://details?id={Application.identifier}");
#endif
}
/// <summary>
/// 앱스토어 리뷰 평가 요청
/// </summary>
private static void RequestAppStoreReview()
{
// 앱스토어 가이드
// 1년에 최대 3번까지 평가를 요청할 수 있습니다.
#if UNITY_IOS
UnityEngine.iOS.Device.RequestStoreReview();
#endif
}
}
예제
private void Start()
{
// 자주 호출되어도 1번만 팝업이 열린다. (클라이언트 파일 저장 기록)
StoreReview.Open();
}
마무리
여러 번 호출이 되어도 1번만 등장합니다. 예외로 장기간 삭제했던 유저가 재설치하는 경우에는 등장할 수 있습니다.
주의하실 점으로는 유저에게 높은 평점을 강요하거나 유도하는 방식으로 진행이된다면 스토어 정책 위반에 해당되기 때문에 자연스러운 흐름에서 만족도가 높은 시점에 호출하셔야합니다. :)
참고 1: https://developer.android.com/guide/playcore/in-app-review/unity?hl=ko
참고 2: https://docs.unity3d.com/ScriptReference/iOS.Device.RequestStoreReview.html
반응형
'Achaive' 카테고리의 다른 글
Unity, Gradle 버전 빌드 애러 (0) | 2023.08.22 |
---|---|
Unity, AAB 설치 완료 체크 (0) | 2023.06.29 |
SPUM 최적화 처리 (드로우콜, 배칭) (0) | 2023.05.07 |
GitHub의 프로젝트를 SVN으로 연결하기 (0) | 2022.09.04 |
코딩 전용 폰트, 캐스캐디아(Cascadia) (0) | 2022.07.28 |
댓글